|
|
|

|
|
|
|
|
|

|
|
 |
Verification Of Memory
|
|
|
In this example, we verify a simple single port RAM. Of course in real life we really don't get to verify a memory model. |
|
|

|
|
|
As usual our testbench will look as shown in figure below. |
|
|

|
|
|
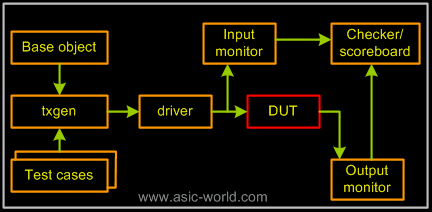 |
|
|

|
|
|
So the verification components are split into following blocks |
|
|

|
|
|
- Base Object
- Transaction Generator
- Driver
- Input Monitor
- Output Monitor
- Scoreboard
- SV testbench top
- Ports File
- HDL Testbench top
|
|
|

|
|
 |
Base Object
|
|
|

|
|
|
1 `ifndef MEM_BASE_OBJECT_SV
2 `define MEM_BASE_OBJECT_SV
3 class mem_base_object;
4 bit [7:0] addr;
5 bit [7:0] data;
6 // Read = 0, Write = 1
7 bit rd_wr;
8 endclass
9 `endif
You could download file sv_examples here
|
|
|

|
|
|

|
|
 |
Transaction Generator
|
|
|

|
|
|
1 `ifndef MEM_TXGEN_SV
2 `define MEM_TXGEN_SV
3 class mem_txgen;
4 mem_base_object mem_object;
5 mem_driver mem_driver;
6
7 integer num_cmds;
8
9 function new(virtual mem_ports ports);
10 begin
11 num_cmds = 3;
12 mem_driver = new(ports);
13 end
14 endfunction
15
16
17 task gen_cmds();
18 begin
19 integer i = 0;
20 for (i=0; i < num_cmds; i ++ ) begin
21 mem_object = new();
22 mem_object.addr = $random();
23 mem_object.data = $random();
24 mem_object.rd_wr = 1;
25 mem_driver.drive_mem(mem_object);
26 mem_object.rd_wr = 0;
27 mem_driver.drive_mem(mem_object);
28 end
29 end
30 endtask
31
32 endclass
33 `endif
You could download file sv_examples here
|
|
|

|
|
|

|
|
|
|
|
|

|
|
 |
Driver
|
|
|

|
|
|
1 `ifndef MEM_DRIVER_SV
2 `define MEM_DRIVER_SV
3
4 class mem_driver;
5 virtual mem_ports ports;
6
7 function new(virtual mem_ports ports);
8 begin
9 this.ports = ports;
10 ports.address = 0;
11 ports.chip_en = 0;
12 ports.read_write = 0;
13 ports.data_in = 0;
14 end
15 endfunction
16
17 task drive_mem (mem_base_object object);
18 begin
19 @ (posedge ports.clock);
20 ports.address = object.addr;
21 ports.chip_en = 1;
22 ports.read_write = object.rd_wr;
23 ports.data_in = (object.rd_wr) ? object.data : 0;
24 if (object.rd_wr) begin
25 $display("Driver : Memory write access-> Address : %x Data : %x\n",
26 object.addr,object.data);
27 end else begin
28 $display("Driver : Memory read access-> Address : %x\n",
29 object.addr);
30 end
31 @ (posedge ports.clock);
32 ports.address = 0;
33 ports.chip_en = 0;
34 ports.read_write = 0;
35 ports.data_in = 0;
36 end
37 endtask
38
39 endclass
40 `endif
41
You could download file sv_examples here
|
|
|

|
|
|

|
|
 |
Input Monitor
|
|
|

|
|
|
1 `ifndef MEM_IP_MONITOR_SV
2 `define MEM_IP_MONITOR_SV
3 class mem_ip_monitor;
4 mem_base_object mem_object;
5 mem_scoreboard sb;
6 virtual mem_ports ports;
7
8 function new (mem_scoreboard sb,virtual mem_ports ports);
9 begin
10 this.sb = sb;
11 this.ports = ports;
12 end
13 endfunction
14
15
16 task input_monitor();
17 begin
18 while (1) begin
19 @ (posedge ports.clock);
20 if ((ports.chip_en == 1) && (ports.read_write == 1)) begin
21 mem_object = new();
22 $display("input_monitor : Memory wr access-> Address : %x Data : %x",
23 ports.address,ports.data_in);
24 mem_object.addr = ports.address;
25 mem_object.data = ports.data_in;
26 sb.post_input(mem_object);
27 end
28 end
29 end
30 endtask
31
32 endclass
33
34 `endif
You could download file sv_examples here
|
|
|

|
|
|

|
|
 |
Output Monitor
|
|
|

|
|
|
1 `ifndef MEM_OP_MONITOR_SV
2 `define MEM_OP_MONITOR_SV
3
4 class mem_op_monitor;
5 mem_base_object mem_object;
6 mem_scoreboard sb;
7 virtual mem_ports ports;
8
9 function new (mem_scoreboard sb,virtual mem_ports ports);
10 begin
11 this.sb = sb;
12 this.ports = ports;
13 end
14 endfunction
15
16
17 task output_monitor();
18 begin
19 while (1) begin
20 @ (negedge ports.clock);
21 if ((ports.chip_en == 1) && (ports.read_write == 0)) begin
22 mem_object = new();
23 $display("Output_monitor : Memory rd access-> Address : %x Data : %x",
24 ports.address,ports.data_out);
25 mem_object.addr = ports.address;
26 mem_object.data = ports.data_out;
27 sb.post_output(mem_object);
28 end
29 end
30 end
31 endtask
32
33
34 endclass
35
36 `endif
You could download file sv_examples here
|
|
|

|
|
|

|
|
 |
Scoreboard
|
|
|

|
|
|
1 `ifndef MEM_SCOREBOARD_SV
2 `define MEM_SCOREBOARD_SV
3
4 class mem_scoreboard;
5 // Create a keyed list to store the written data
6 // Key to the list is address of write access
7 mem_base_object mem_object [*];
8
9 // post_input method is used for storing write data
10 // at write address
11 task post_input (mem_base_object input_object);
12 begin
13 mem_object[input_object.addr] = input_object;
14 end
15 endtask
16 // post_output method is used by the output monitor to
17 // compare the output of memory with expected data
18 task post_output (mem_base_object output_object);
19 begin
20 // Check if address exists in scoreboard
21 if (mem_object[output_object.addr] ! = null) begin
22 mem_base_object in_mem = mem_object[output_object.addr];
23 $display("scoreboard : Found Address %x in list",output_object.addr);
24 if (output_object.data ! = in_mem.data) begin
25 $display ("Scoreboard : Error : Exp data and Got data don't match");
26 $display(" Expected -> %x",
27 in_mem.data);
28 $display(" Got -> %x",
29 output_object.data);
30 end else begin
31 $display("Scoreboard : Exp data and Got data match");
32 end
33 end
34 end
35 endtask
36
37 endclass
38
39 `endif
You could download file sv_examples here
|
|
|

|
|
|

|
|
|

|
|
|

|
|
|
|
|
|

|
|
|
|
|
|

|
|
Copyright © 1998-2025 |
Deepak Kumar Tala - All rights reserved |
Do you have any Comment? mail me at:deepak@asic-world.com
|
|