|
|

|
|
|
|
|
|

|
|
 |
C Data Types
|
|
|
C has a concept of 'data types' which are used to define a variable before its use. |
|
|

|
|
|
The definition of a variable will assign storage for the variable and define the type of data that will be held in the location. |
|
|

|
|
|
Following are the data types available in C language. |
|
|

|
|
|
- int : integer variable
- short : short integer
- long : long integer
- float : single precision real (floating point) variable
- double : double precision real (floating point) variable
- char : character variable (single byte)
- void : variable without any type.
- enum : enumerated data type.
|
|
|

|
|
|
The printf function can be instructed to print integers, floats and strings properly. The general syntax is |
|
|

|
|
|
printf( "format", variables ); |
|
|

|
|
|
where "format" specifies the converstion specification and variables is a list of quantities to print. Some useful formats are |
|
|

|
|
|
- %.nd integer (optional n = number of columns; if 0, pad with zeroes)
- %m.nf float or double (optional m = number of columns, n = number of decimal places)
- %ns string (optional n = number of columns)
- %c character
- \n \t to introduce new line or tab
- \g ring the bell (``beep'') on the terminal
|
|
|

|
|
 |
int - data type
|
|
|
int is used to define integer numbers. |
|
|

|
|
|
1 {
2 int Count;
3 Count = 5;
4 }
You could download file int.c here
|
|
|

|
|
 |
short- data type
|
|
|
short is used to define integer numbers. |
|
|

|
|
|
1 {
2 short Count;
3 Count = 5;
4 }
You could download file short.c here
|
|
|

|
|
 |
long- data type
|
|
|
long is used to define integer numbers. |
|
|

|
|
|
1 {
2 long Count;
3 Count = 5;
4 }
You could download file long.c here
|
|
|

|
|
 |
float - data type
|
|
|
float is used to define floating point numbers. |
|
|

|
|
|
1 {
2 float Miles;
3 Miles = 5.6;
4 }
You could download file float.c here
|
|
|

|
|
 |
double - data type
|
|
|
double is used to define BIG floating point numbers. It reserves twice the storage for the number. On PCs this is likely to be 8 bytes. |
|
|

|
|
|
1 {
2 double Atoms;
3 Atoms = 2500000;
4 }
You could download file double.c here
|
|
|

|
|
|
|
|
|

|
|
 |
char - data type
|
|
|
char defines characters. |
|
|

|
|
|
1 {
2 char Letter;
3 Letter = 'x';
4 }
You could download file char.c here
|
|
|

|
|
 |
void
|
|
|
The void keyword allows us to create functions that either do not require any parameters or do not return a value. |
|
|

|
|
|
1 #include <stdio.h>
2
3 void Print_Square(int Number);
4
5 void main ()
6 {
7 Print_Square(5);
8 exit(0);
9 }
10
11 void Print_Square(int Number)
12 {
13 printf("%d squared is %d\n",Number, Number*Number);
14 }
You could download file void.c here
|
|
|

|
|
 |
enum
|
|
|
ENUM allows you to define a list of aliases which represent integer numbers. |
|
|

|
|
|
1 #include <stdio.h>
2
3 enum boolean {FALSE=0, TRUE };
4 enum months {Jan=5, Feb, Mar, Apr, May, Jun, Jul, Aug, Sep, Oct, Nov, Dec};
5
6 void main() {
7 enum months month;
8 enum boolean mybool;
9 printf("Month %d\n", month=Aug);
10 printf("Bool %d\n", mybool=TRUE);
11 }
You could download file enum.c here
|
|
|

|
|
 |
Global Variables
|
|
|
Local variables are declared within the body of a function, and can only be used within that function. This is usually no problem, since when another function is called, all required data is passed to it as arguments. Alternatively, a variable can be declared globally so it is available to all functions. Modern programming practice recommends against the excessive use of global variables. They can lead to poor program structure, and tend to clog up the available name space. |
|
|

|
|
|
A global variable declaration looks normal, but is located outside any of the program's functions. This is usually done at the beginning of the program file, but after preprocessor directives. The variable is not declared again in the body of the functions which access it. |
|
|

|
|
 |
Static Variables
|
|
|
Another class of local variable is the static type. A static can only be accessed from the function in which it was declared, like a local variable. The static variable is not destroyed on exit from the function, instead its value is preserved, and becomes available again when the function is next called. Static variables are declared as local variables, but the declaration is preceeded by the word static. |
|
|

|
|
|
static int counter; |
|
|

|
|
|
Static variables can be initialised as normal, the initialisation is performed once only, when the program starts up. |
|
|

|
|
 |
External Variables
|
|
|
Where a global variable is declared in one file, but used by functions from another, then the variable is called an external variable in these functions, and must be declared as such. The declaration must be preceeded by the word extern. The declaration is required so the compiler can find the type of the variable without having to search through several source files for the declaration. |
|
|

|
|
|
Global and external variables can be of any legal type. They can be initialised, but the initialisation takes place when the program starts up, before entry to the main function. |
|
|

|
|
 |
Arrays
|
|
|
Arrays of any type can be formed in C. The syntax is simple |
|
|

|
|
|
type name[dim]; |
|
|

|
|
|
Arrays can be created from any of the C data types int, float, char. I start with int and float as these are the easiest to understand. Then move onto int and float multi dimentional arrays and finally char arrays |
|
|

|
|
|
In C, arrays starts at position 0. The elements of the array occupy adjacent locations in memory. C treats the name of the array as if it were a pointer to the first element--this is important in understanding how to do arithmetic with arrays. Thus, if v is an array, *v is the same thing as v[0], *(v+1) is the same thing as v[1], and so on. |
|
|

|
|
|
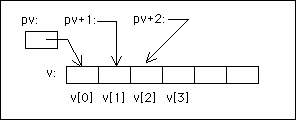 |
|
|

|
|
|
1 #define SIZE 3
2
3 void main() {
4 float x[SIZE];
5 float *fp;
6 int i;
7 // initialize the array x use a "cast" to force i into the equivalent float
8 for (i = 0; i < SIZE; i++) {
9 x[i] = 0.5*(float)i;
10 }
11 for (i = 0; i < SIZE; i++) {
12 printf(" %d %f \n", i, x[i]);
13 }
14 // make fp point to array x
15 fp = x;
16 // print via pointer arithmetic members of x are adjacent to
17 // each other in memory *(fp+i) refers to content of
18 // memory location (fp+i) or x[i]
19 for (i = 0; i < SIZE; i++) {
20 printf(" %d %f \n", i, *(fp+i));
21 }
22 }
You could download file array.c here
|
|
|

|
|
|

|
|
|

|
|
|
|
|
|

|